The Migration Guide intends to guide users who already have experience with CC3200 devices and need to port their software to CC3220x/CC3235x family of devices. The document describes the SDK structure and highlights the software components that require attention including host driver, OS layers, board drivers, and external libraries. This is v1.5.0 of the CC3200 SDK. Getting Started. To get started using the CC3200 LaunchPad with the Out of Box experience, please see the CC3200 Quick Start Guide. To get started with your project development, please see the CC3200 Getting Started Guide. New APIs added to the host driver. API to mask supported rates in the RX and TX. This is a starter project for the Texas Instruments CC3200 LaunchPad using TI-RTOS - remixed123/startprojectcc3200ti-rtos.
CC3200 HTTP Client Project
http-client C-source code
Project on Hackaday
http_client_demo project - remotely view your CC3200 data and control LED at http://cnktechlabs.com/webapp_pub/grid.php
I have developed a simple web application for the CC3200 development board, an AK9753 Human Presence Sensor Breakout board , and an on-board TMP006 sensor.
The web application displays data collected from all sensors and allows to switch (ON/OFF) the on-board Red LED. I am planning to add more functions to the web application.
Show below is the image of my current circuit wiring diagram. The MB1212 Maxbotix sensors will be used as motion sensors in the project.
The CC3200 LaunchPad development board has an on-board temperature sensor (TMP006-Infrared Thermopile Sensor in Chip-Scale Package) and an accelerometer. Both sensors use I2C bus for data transfer.
A note on the AK9753 sensor
The AK9753 is a low power and compact infrared-ray (IR) sensor module. It is composed of four quantum IR sensors and an integrated circuit (IC) for characteristic compensation. The four IR sensors' offset and gain variations are calibrated at shipment. An integral analog-to-digital converter provides 16-bits data outputs. The AK9753 is suitable for several feet human detector by using external lens.
The AK9753 datasheet link.
Click on the image below to view the web-app:
Cc3200 Sdk For Mac Installer
To run a quick test of the project with your CC3200 development board:
- Download and install the CC3200 SDK on your PC.
- Download C-source code files from the Github. One of the files listed on the Github is a zip file of the CCS project (CC3200_client(CCS-project).zip).
You can download and unzip the file. - Connect CC3200 development board to a computer.
- Open a serial terminal on your PC and Set: COM PORT X, 115200 bps, 8N1, no parity, no flow control.
- I recommend (it is not required) changing the MAC address from a default one. The MAC address will be used as a unique ID number to monitor data sent by your board. To set a new MAC address enter unique values as shown below MAC_Address[0] .. MAC_Address[5]. After the board is programmed you will need to press and hold SW3 and push the Reset button while pressing SW3. Afetr a 10 - 15 seconds you see ' MAC address is set to: XX:XX:XX:XX:XX:XX' printen on a serial terminal:
- Enter your WiFi credentials (SSID name and password) in the common.h file:
- Save your project with the new credentials and compile it.
- Once the project is compiled the 'CC3200_client_web.bin' will be located in the Release folder:
- Program the CC3200 board with the CCS uniflash.
- If you want to change the MAC address from the default one, press and hold SW3 and push the reset button. Keep SW3 pressed untill the new MAC address is set and printed. This may take 15-20 sec
- Write down the number shown below. This is the ID number of your board. You will need it to display your data on the web-page. Conver the HEX value to a decimal and enter it on the web-app page as the ID number:
- Monitor and control CC3200 board from cnktechlabs.com/webapp_pub/grid.php.
- Enter the ID number in a decimal format:
The main.c file:
The buffer 'buf' is loaded below with all the information that will be sent to the cloud:
HTTP POST method is used here to send data to a server to create/update a resource.
Project Details:
The C-source code has beed updated. The new code allows remote control and monitoring of the CC3200 board with AK9753 (Qwiic) sensor connected to I2C port. The SparkFun AK9753 Human Presence Sensor Breakout is a Qwiic-enabled, 4-channel Nondispersive Infrared (NDIR) sensor. The board reports data collected from the AK9753 Human Presence sensor to the grid.php webpage, the onboard TMP006 I2C digital temperature sensor, and current status of the Red LED. The same webpage can be used to turn ON or OFF the onboard red LED. AK9753 is connected to I2C lines on the board - PIN_01 is the SCL and PIN_02 is the SDA. Compile and download CC3200_client.bin file into CC3200 development board. Connect Dev board to a PC. View USB messages on a Serial terminal on your PC. Monitor status of CC3200 sensors and Red LED as well as control the Red LED from cnktechlabs.com/webapp_pub/grid.php webpage.
How the data is transmitted and received over the web:
In the main file there are two functions:
- HTTPPostMethod_data(&httpClient);
- HTTPGetPageMethod(&httpClient);
They are used to transmit data to, and receive data from the grid.php webpage. The first function posts data to the grid.php web-page approximately once a minute.
The second function from the list receives commands from the grid.php web-page every 4-5 seconds.
The data that is sent over the web link is collected in the char buf[99] buffer:
Inside the buffer the data string looks as follows:
IR1=644 & IR2=494 & IR3=410 & IR4=562 & RoomT=22.90 & TName=controllall
The following code snippet reads current state of the Red LED and saves it in the buf buffer.
Once all data is collected it is sent over the Wi-Fi link.The HTTPPostMethod_data will send data:
static int HTTPPostMethod_data(HTTPCli_Handle httpClient);
Inside the HTTPPostMethod_data funtion HTTPCli_sendRequest will built a HTTP request:
lRetVal = HTTPCli_sendRequest(httpClient, HTTPCli_METHOD_POST, POST_REQUEST_URI, moreFlags);
lRetVal = HTTPCli_sendRequestBody(httpClient, buf, (sizeof(buf)-14)) function will send data saved in buf over the web.
The data is also streamed over the USB cabel to a local PC:
Project Start:
Recently I started tinkering with the http_client_demo CC3200 example project. The code demonstrates different HTTP web services methods: like GET, POST, PUT, and DELETE. My goal is to write a code that will connect CC3200 wireless MCU board to my website and send temperature and accelerometer data over the internet.
I am going to start with the GET method and then continue with the POST method.
The GET method
The GET method sends URL page as well as data in a single string. The page and the encoded information are separated by the ? character.
http://www.cnktechlabs.com/get.php?name1=value1&name2=value2
The text that follows the ? is the query string.
The GET method is restricted to send up to 1024 characters only.
To test the GET method I have created and uploaded /get.php PHP file to my website (www.cnktechlabs.com/get.php).
String sent by CC3200 to www.cnktechlabs.com - 'www.cnktechlabs.com/get.php?id=goodbye&mode=run'
Below is the php code for the get.php page.
To get a response from a web page with the PHP code shown above I send the following string (main.c):
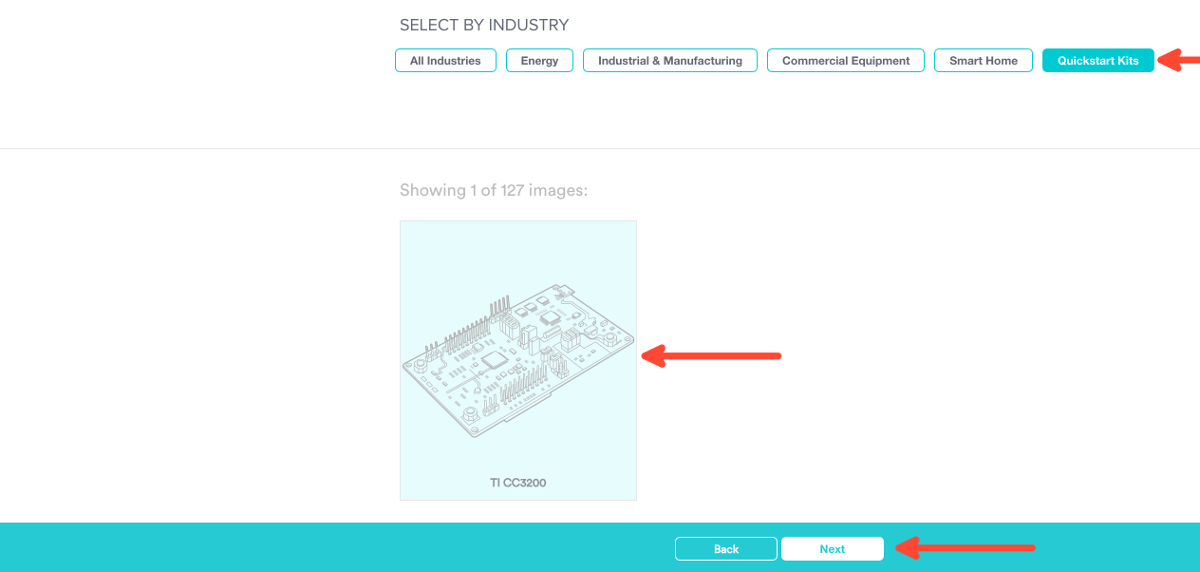
This is how I send a request to www.cnktechlabs.com/get.php file:
GET(parameters) -----------------------> www.cnktechlabs.com/get.php?id=goodbye&mode=run'
The web page get.php receives the data sent by the http client (CC3200 development) board and echoes it back.
Response from www.cnktechlabs.com/get.php file:
CC3200 (the response is printed on a serial interface) <---------- www.cnktechlabs.com/get.php
Mere brother ki dulhan cast. Response from the server printed on a serial terminal:
As you can see from the above printout the get.php page replies with page content that includes punctuation marks and html tags.
Using the GET method from the http_client_demo.c code example:
The data is appended to the URL as a series of name/value pairs. After the URL web address has ended, we include a question mark (?) followed by the name/value pairs, each one separated by an ampersand (&).
Image below shows the response from the get.php webpage printer on 'Termite' serial terminal.
As you can see from above image the get.php responds with html tags and text inside the tags, as well as text echoed from the PHP code.POST method
A POST request passes parameters in the body of the HTTP request, leaving the URL untouched. I have uploaded the following file to my server - www.cnktechlabs.com/post.php:
post.php
The POST method implementation with CC3200 Code:
Server response:
Hi Mom
The next step is to collect and send some data to a webpage. I have created post.php and data.html webpages to collect and display data. The 'post.php' file shown below is responsible for reading data from the CC3200 board.
main.c:
HTTPPostMethod_data function, shown below, will send temperature, accelerometer, and location data to the post.php file.
Below is the result I can view on my data.html webpage.
Image of the serial print out on the Termite Terminal
Below is the result I can view on my data.html webpage.
A new hardware addition to the project - Light Sensor
Light Sensor senses ambient light and transmits messages to the web page. The data out, DO, pin of the sensor is connected to GPIO_03, PIN_58 on the development board.
Current Project Circuit Diagram
Project Source Code
Settings for the serial port: 115200, 8N1, no handshake.
Enter PASSWORD and SSID name in the 'common.h' file
Change location (strPtr = ' & loc=Los Angeles 0'; // Your location.) to your geographical location in the source code main file.
Flush the project into your CC3200 board, and view the results on the www.cnktechlabs.com/data.html. Nissan consult software download. Connect CC3200 to a serial terminal on your PC.
Reset the board.
To enter password and SSID name either:
Press and hold SW2 and reset the board
On prompt 'Enter Password' enter WiFi password
On prompt 'SSID name' enter WiFi name.
Or just reset the board and the common.h credentials will be used.
Introduction
The SimpleLink™ Wi-Fi® CC32xx device is a single-chip microcontroller (MCU) with built-in Wi-Fi connectivity, created for the Internet of Things (IoT). The CC32xx device is a wireless MCU that integrates a high-performance ARM® Cortex®-M4 MCU, allowing customers to develop an entire application with a single IC.
The Texas Instruments royalty-free CC32xx Embedded Wi-Fi foundation software development kit (SDK) is a complete software platform for developing Wi-Fi applications. It is based on the CC3220 and cc3235 devices, a complete Wi-Fi SoC (System-on-Chip) solution. The CC32xx family of devices comes in following variants:
- CC3220 – Single-Band 2.4GHz Wi-Fi, MCU with 256KB of RAM, IoT networking security and device identity/keys
- CC3220S - Single-Band 2.4GHz Wi-Fi, MCU with 256KB of RAM, IoT networking security, device identity/keys as well as MCU level security such as file system encryption, user IP (MCU image) encryption, secure boot and debug security
- CC3220SF - Single-Band 2.4GHz Wi-Fi, MCU with 1MB user-dedicated flash and 256KB of RAM, IoT networking security, device identity/keys as well as MCU level security such as file system encryption, user IP (MCU image) encryption, secure boot and debug
- CC3235S – Dual-Band 2.4 and 5-GHz Wi-Fi, MCU with 256KB of RAM, IoT networking security, device identity/keys as well as MCU level security such as file system encryption, user IP (MCU image) encryption, secure boot and debug security
- CC3235SF - Dual-Band 2.4 and 5-GHz Wi-Fi, MCU with 1MB user-dedicated flash and 256KB of RAM, IoT networking security, device identity/keys as well as MCU level security such as file system encryption, user IP (MCU image) encryption, secure boot and debug
The SimpleLink™ Wi-Fi® CC32xx SDK contains drivers, libraries, example code for the CC32xx programmable MCU and documentation needed to use the solution. It also contains the flash programmer, a command line tool for flashing software, configuring network and software parameters (SSID, access point channel, network profile, etc.), system files, and user files (certificates, web pages, etc). This SDK can be used with TI's SimpleLink Wi-Fi CC32xx LaunchPads.
The CC32xx solution combines a 2.4-GHz and 5-Ghz Wi-Fi PHY/MAC and TCP/IP networking engine with a microcontroller, up to 256-kB on-chip RAM, 1-MB internal flash (for CC32xx SF), and a comprehensive range of peripherals.
The SimpleLink™ MCU portfolio offers a single development environment that delivers flexible hardware, software and tool options for customers developing wired and wireless applications. With 100 percent code reuse across host MCUs, Wi-Fi™, Bluetooth® low energy, Sub-1GHz devices and more, choose the MCU or connectivity standard that fits your design. A one-time investment with the SimpleLink software development kit (SDK) allows you to reuse often, opening the door to create unlimited applications. For more information, visit www.ti.com/simplelink.
This is version 4_30_00_06 of the SimpleLink CC32xx SDK.
Documentation
What's New
- Wi-Fi Certified WPA3 support and Network Assisted Roaming feature on CC3x3x
- WPA3 in Station role only
- WPA3 support includes personal and enterprise (128 Bit key) support
- WPA3 support on CC3x20
- Station role only
- Includes personal and enterprise (128 Bit key) support
- Introduces Connection Manager application module
- Simplifies the interface for setting up MAC, IP or Internet level connection using the SimpleLink Network (SlNet) Framework
- Enables easy integration of the SimpleLink Wi-Fi provisioning
- Adds new example application which is an alternative to the provisioning example. Demonstrates the use of the connection manager through the first connection to the network using the SimpleLink provisioning and supports reconnecting based on a stored profile.
- Adds support for SysConfig GenLibs feature - once adding a module through syscfg, it adds the relevant .a lib file into the project automatically
- Adds support for TI Clang compiler to examples
- Beacon tracking algorithm enhancements (power optimization)
- Fixed some security issues in CC3x3x SP, Downgrade protection is applied for this version (4.8.0.8) and cannot be downgraded
- SysConfig Logging feature included in beta mode
- See the beta disclaimer in the tiutils/log SDK example readme
- TI Utils Execution Graph feature is not suggested for customer use for this release
- Bug fixes
- Please refer to the Documentation Overview for the details about each SDK component
- SimpleLink Platform level changes are available in SimpleLink Core SDK Release Notes
- SDK Change Log provides a list of all SDK component changes
Updating service-pack
It is recommended to update the service pack to the latest available, which can be found in the following path:
CC3220 Service pack - /tools/cc32xx_tools/servicepack-cc3x20
CC3235 Service pack - /tools/cc32xx_tools/servicepack-cc3x35
CC3220 NWP Service Pack Release Notes.
CC3235 NWP Service Pack Release Notes.
For more information please follow the instructions in the Quick start Guide.
Upgrade and Compatibility Information
The Migration Guide intends to guide users who already have experience with CC3200 devices and need to port their software to CC3220x/CC3235x family of devices. The document describes the SDK structure and highlights the software components that require attention including host driver, OS layers, board drivers, and external libraries.
Operating Systems Support
- Windows 7, Windows 8, Windows 10
- Ubuntu
- Mac OS X
Dependencies
This release was validated with the following components:
- Code Composer Studio 10.1.1
- ARM 20.2.1.LTS
- XDCTools 3.61.02.27
- GNU Code Generation Tools
- IAR Code Generation Tools
- TI-Clang 1.0.0 STS
- SimpleLink host driver 3.0.1.68
Device Support
- CC3220R: use CC3220S examples from CC3220S_LAUNCHXL folders (mcu image security steps not needed)
- CC3220S: use CC3220S examples from CC3220S_LAUNCHXL folders
- CC3220SF: use CC3220SF examples from CC3220SF_LAUNCHXL folders
- CC3235S: use CC3235S examples from CC3235S_LAUNCHXL folders
- CC3235SF: use CC3235SF examples from CC3235SF_LAUNCHXL folders
Known Issues
ID | Summary |
---|---|
CC3X20SDK-1898 | Network terminal: radiotool fails to start transceiver mode |
CC3X20SDK-1600 | Network terminal - Radio tool, Can be set device that supports 2.4GHz only, on 5G channels. |
CC3X20SDK-1569 | Calling sl_Stop during sl_Select may cause assert |
CC3X20SDK-1527 | AT Commands: Http client read response body command with length larger than 900 bytes might cause memory allocation fault |
CC3X20SDK-1525 | MQTT Client: connection with user name and without password is not functional |
CC3X20SDK-1514 | Cloud OTA :OTA TAR with name larger than 109 characters stops the OTA process without an error |
CC3X20SDK-1467 | Network terminal - issue parsing IP address in wlancommand |
CC3X20SDK-1450 | OTA Lib: wrong handling of fragmented HTTP response |
CC3X20SDK-1423 | AT Commands: MDNS service is not advertised when flag is IPV6_IPV4_ONLY used, need to add IS_UNIQUE_BIT flag in order to make it advertise |
CC3X20SDK-1376 | AT Commands: transceiver with incorrect channel in AT command causes Health monitor assert |
CC3X20SDK-601 | Rarely the MQTT Server internal bridging does not work from server to client |
CC3X20SDK-156 | Rarely the progress bar get stuck during OTA procedure |
CC3X20SDK-116 | Progress bar on Windows FireFox and Chrome and Chrome browser doesn't update process status on the fly |
Versioning
This product's version follows a version format, M.mm.pp.bb, where M is a single digit Major number, mm is 2 digit minor number, pp is a 2 digit patch number, and b is an unrestricted set of digits used as an incrementing build counter.
M - Is incremented for the first release every year. 1 -> 2017, 2 -> 2018, and so on.
Cc3200 Sdk For Mac Windows 7
mm - indicates the specific quarter of the year the SDK was released. 10 -> Q1; 20 -> Q2; 30 -> Q3; 40 -> Q4
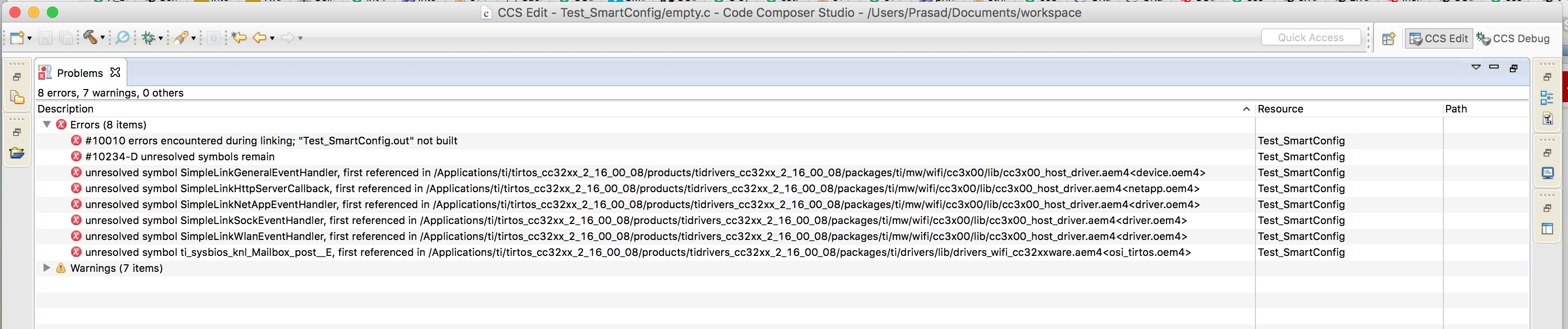
This is how I send a request to www.cnktechlabs.com/get.php file:
GET(parameters) -----------------------> www.cnktechlabs.com/get.php?id=goodbye&mode=run'
The web page get.php receives the data sent by the http client (CC3200 development) board and echoes it back.
Response from www.cnktechlabs.com/get.php file:
CC3200 (the response is printed on a serial interface) <---------- www.cnktechlabs.com/get.php
Mere brother ki dulhan cast. Response from the server printed on a serial terminal:
As you can see from the above printout the get.php page replies with page content that includes punctuation marks and html tags.
Using the GET method from the http_client_demo.c code example:
The data is appended to the URL as a series of name/value pairs. After the URL web address has ended, we include a question mark (?) followed by the name/value pairs, each one separated by an ampersand (&).
Image below shows the response from the get.php webpage printer on 'Termite' serial terminal.
As you can see from above image the get.php responds with html tags and text inside the tags, as well as text echoed from the PHP code.POST method
A POST request passes parameters in the body of the HTTP request, leaving the URL untouched. I have uploaded the following file to my server - www.cnktechlabs.com/post.php:
post.php
The POST method implementation with CC3200 Code:
Server response:
Hi Mom
The next step is to collect and send some data to a webpage. I have created post.php and data.html webpages to collect and display data. The 'post.php' file shown below is responsible for reading data from the CC3200 board.
main.c:
HTTPPostMethod_data function, shown below, will send temperature, accelerometer, and location data to the post.php file.
Below is the result I can view on my data.html webpage.
Image of the serial print out on the Termite Terminal
Below is the result I can view on my data.html webpage.
A new hardware addition to the project - Light Sensor
Light Sensor senses ambient light and transmits messages to the web page. The data out, DO, pin of the sensor is connected to GPIO_03, PIN_58 on the development board.
Current Project Circuit Diagram
Project Source Code
Settings for the serial port: 115200, 8N1, no handshake.
Enter PASSWORD and SSID name in the 'common.h' file
Change location (strPtr = ' & loc=Los Angeles 0'; // Your location.) to your geographical location in the source code main file.
Flush the project into your CC3200 board, and view the results on the www.cnktechlabs.com/data.html. Nissan consult software download. Connect CC3200 to a serial terminal on your PC.
Reset the board.
To enter password and SSID name either:
Press and hold SW2 and reset the board
On prompt 'Enter Password' enter WiFi password
On prompt 'SSID name' enter WiFi name.
Or just reset the board and the common.h credentials will be used.
Introduction
The SimpleLink™ Wi-Fi® CC32xx device is a single-chip microcontroller (MCU) with built-in Wi-Fi connectivity, created for the Internet of Things (IoT). The CC32xx device is a wireless MCU that integrates a high-performance ARM® Cortex®-M4 MCU, allowing customers to develop an entire application with a single IC.
The Texas Instruments royalty-free CC32xx Embedded Wi-Fi foundation software development kit (SDK) is a complete software platform for developing Wi-Fi applications. It is based on the CC3220 and cc3235 devices, a complete Wi-Fi SoC (System-on-Chip) solution. The CC32xx family of devices comes in following variants:
- CC3220 – Single-Band 2.4GHz Wi-Fi, MCU with 256KB of RAM, IoT networking security and device identity/keys
- CC3220S - Single-Band 2.4GHz Wi-Fi, MCU with 256KB of RAM, IoT networking security, device identity/keys as well as MCU level security such as file system encryption, user IP (MCU image) encryption, secure boot and debug security
- CC3220SF - Single-Band 2.4GHz Wi-Fi, MCU with 1MB user-dedicated flash and 256KB of RAM, IoT networking security, device identity/keys as well as MCU level security such as file system encryption, user IP (MCU image) encryption, secure boot and debug
- CC3235S – Dual-Band 2.4 and 5-GHz Wi-Fi, MCU with 256KB of RAM, IoT networking security, device identity/keys as well as MCU level security such as file system encryption, user IP (MCU image) encryption, secure boot and debug security
- CC3235SF - Dual-Band 2.4 and 5-GHz Wi-Fi, MCU with 1MB user-dedicated flash and 256KB of RAM, IoT networking security, device identity/keys as well as MCU level security such as file system encryption, user IP (MCU image) encryption, secure boot and debug
The SimpleLink™ Wi-Fi® CC32xx SDK contains drivers, libraries, example code for the CC32xx programmable MCU and documentation needed to use the solution. It also contains the flash programmer, a command line tool for flashing software, configuring network and software parameters (SSID, access point channel, network profile, etc.), system files, and user files (certificates, web pages, etc). This SDK can be used with TI's SimpleLink Wi-Fi CC32xx LaunchPads.
The CC32xx solution combines a 2.4-GHz and 5-Ghz Wi-Fi PHY/MAC and TCP/IP networking engine with a microcontroller, up to 256-kB on-chip RAM, 1-MB internal flash (for CC32xx SF), and a comprehensive range of peripherals.
The SimpleLink™ MCU portfolio offers a single development environment that delivers flexible hardware, software and tool options for customers developing wired and wireless applications. With 100 percent code reuse across host MCUs, Wi-Fi™, Bluetooth® low energy, Sub-1GHz devices and more, choose the MCU or connectivity standard that fits your design. A one-time investment with the SimpleLink software development kit (SDK) allows you to reuse often, opening the door to create unlimited applications. For more information, visit www.ti.com/simplelink.
This is version 4_30_00_06 of the SimpleLink CC32xx SDK.
Documentation
What's New
- Wi-Fi Certified WPA3 support and Network Assisted Roaming feature on CC3x3x
- WPA3 in Station role only
- WPA3 support includes personal and enterprise (128 Bit key) support
- WPA3 support on CC3x20
- Station role only
- Includes personal and enterprise (128 Bit key) support
- Introduces Connection Manager application module
- Simplifies the interface for setting up MAC, IP or Internet level connection using the SimpleLink Network (SlNet) Framework
- Enables easy integration of the SimpleLink Wi-Fi provisioning
- Adds new example application which is an alternative to the provisioning example. Demonstrates the use of the connection manager through the first connection to the network using the SimpleLink provisioning and supports reconnecting based on a stored profile.
- Adds support for SysConfig GenLibs feature - once adding a module through syscfg, it adds the relevant .a lib file into the project automatically
- Adds support for TI Clang compiler to examples
- Beacon tracking algorithm enhancements (power optimization)
- Fixed some security issues in CC3x3x SP, Downgrade protection is applied for this version (4.8.0.8) and cannot be downgraded
- SysConfig Logging feature included in beta mode
- See the beta disclaimer in the tiutils/log SDK example readme
- TI Utils Execution Graph feature is not suggested for customer use for this release
- Bug fixes
- Please refer to the Documentation Overview for the details about each SDK component
- SimpleLink Platform level changes are available in SimpleLink Core SDK Release Notes
- SDK Change Log provides a list of all SDK component changes
Updating service-pack
It is recommended to update the service pack to the latest available, which can be found in the following path:
CC3220 Service pack - /tools/cc32xx_tools/servicepack-cc3x20
CC3235 Service pack - /tools/cc32xx_tools/servicepack-cc3x35
CC3220 NWP Service Pack Release Notes.
CC3235 NWP Service Pack Release Notes.
For more information please follow the instructions in the Quick start Guide.
Upgrade and Compatibility Information
The Migration Guide intends to guide users who already have experience with CC3200 devices and need to port their software to CC3220x/CC3235x family of devices. The document describes the SDK structure and highlights the software components that require attention including host driver, OS layers, board drivers, and external libraries.
Operating Systems Support
- Windows 7, Windows 8, Windows 10
- Ubuntu
- Mac OS X
Dependencies
This release was validated with the following components:
- Code Composer Studio 10.1.1
- ARM 20.2.1.LTS
- XDCTools 3.61.02.27
- GNU Code Generation Tools
- IAR Code Generation Tools
- TI-Clang 1.0.0 STS
- SimpleLink host driver 3.0.1.68
Device Support
- CC3220R: use CC3220S examples from CC3220S_LAUNCHXL folders (mcu image security steps not needed)
- CC3220S: use CC3220S examples from CC3220S_LAUNCHXL folders
- CC3220SF: use CC3220SF examples from CC3220SF_LAUNCHXL folders
- CC3235S: use CC3235S examples from CC3235S_LAUNCHXL folders
- CC3235SF: use CC3235SF examples from CC3235SF_LAUNCHXL folders
Known Issues
ID | Summary |
---|---|
CC3X20SDK-1898 | Network terminal: radiotool fails to start transceiver mode |
CC3X20SDK-1600 | Network terminal - Radio tool, Can be set device that supports 2.4GHz only, on 5G channels. |
CC3X20SDK-1569 | Calling sl_Stop during sl_Select may cause assert |
CC3X20SDK-1527 | AT Commands: Http client read response body command with length larger than 900 bytes might cause memory allocation fault |
CC3X20SDK-1525 | MQTT Client: connection with user name and without password is not functional |
CC3X20SDK-1514 | Cloud OTA :OTA TAR with name larger than 109 characters stops the OTA process without an error |
CC3X20SDK-1467 | Network terminal - issue parsing IP address in wlancommand |
CC3X20SDK-1450 | OTA Lib: wrong handling of fragmented HTTP response |
CC3X20SDK-1423 | AT Commands: MDNS service is not advertised when flag is IPV6_IPV4_ONLY used, need to add IS_UNIQUE_BIT flag in order to make it advertise |
CC3X20SDK-1376 | AT Commands: transceiver with incorrect channel in AT command causes Health monitor assert |
CC3X20SDK-601 | Rarely the MQTT Server internal bridging does not work from server to client |
CC3X20SDK-156 | Rarely the progress bar get stuck during OTA procedure |
CC3X20SDK-116 | Progress bar on Windows FireFox and Chrome and Chrome browser doesn't update process status on the fly |
Versioning
This product's version follows a version format, M.mm.pp.bb, where M is a single digit Major number, mm is 2 digit minor number, pp is a 2 digit patch number, and b is an unrestricted set of digits used as an incrementing build counter.
M - Is incremented for the first release every year. 1 -> 2017, 2 -> 2018, and so on.
Cc3200 Sdk For Mac Windows 7
mm - indicates the specific quarter of the year the SDK was released. 10 -> Q1; 20 -> Q2; 30 -> Q3; 40 -> Q4